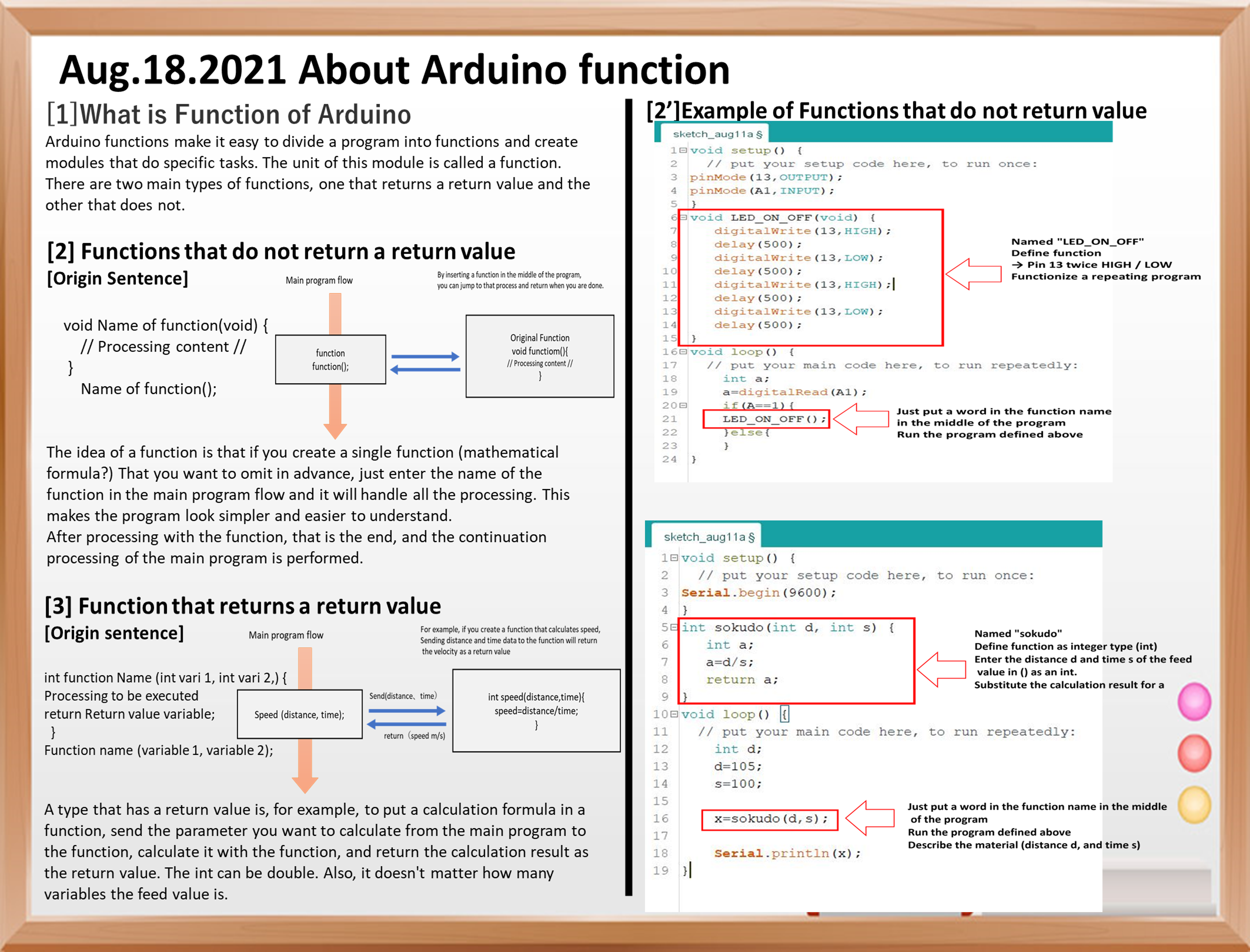
This time, I will talk about Arduino’s Void function.
Arduino functions make it easy to divide a program into functions and create modules that do specific tasks. The unit of this module is called a function.
There are two main types of
functions, one that returns a return value and the other that does not.
Functions that do not return a return value
First, the type of program that does not return a return value is shown below.
void name of function(void) {
//Processing content//
}
name of function();
A function that does not return a return value is used when you want the function to do only some work and the work result is not used in the sketch. In some cases, information is passed to a function (called an argument) to make it work.
The idea of a function is that if you create a single function (mathematical formula?) That you want to omit in advance, just enter the name of the function in the main program flow and the process will go through. It does the job, making the program look simpler and easier to understand.
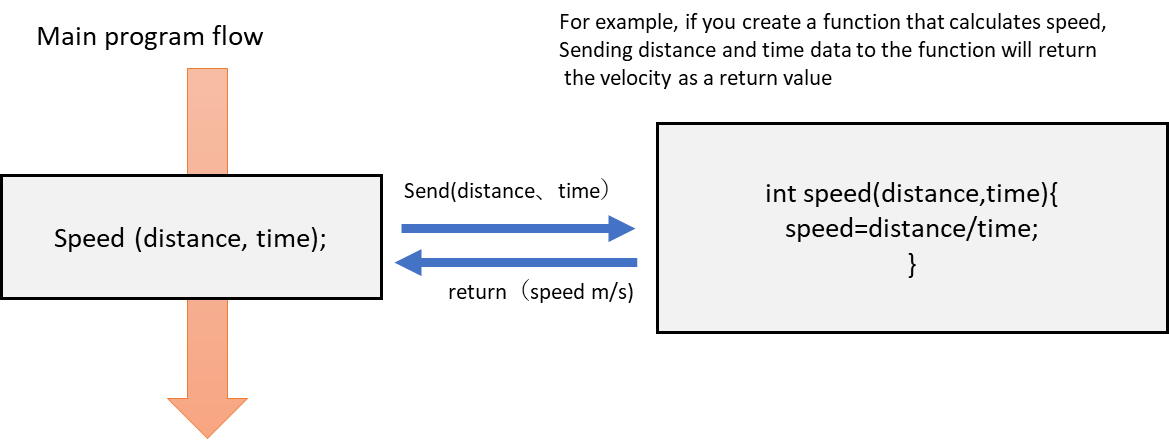
The figure below is an example of the program.
void setup() {
// put your setup code here, to run once:
pinMode(13,OUTPUT);
pinMode(A1,INPUT);
}
void LED_ON_OFF(void) {
digitalWrite(13,HIGH);
delay(500);
digitalWrite(13,LOW);
delay(500);
digitalWrite(13,HIGH);
delay(500);
digitalWrite(13,LOW);
delay(500);
}
void loop() {
// put your main code here, to run repeatedly:
int a;
a=digitalRead(A1);
if(A==1){
LED_ON_OFF();
}else{
}
}
A diagram summarizing this is shown below.
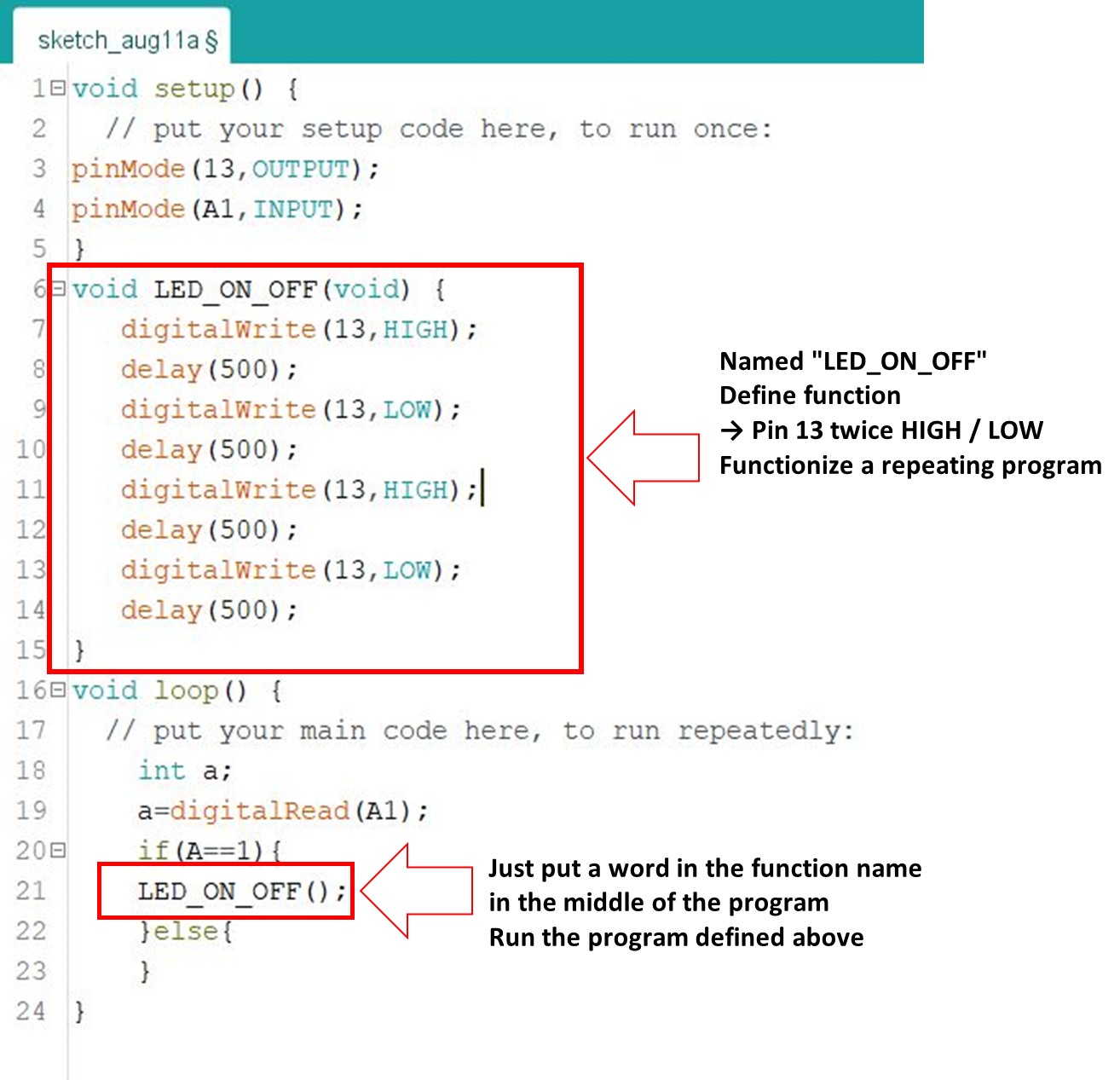
The program that turns the LED on / off with void LED_ON_OFF (void) {processing} is made into a function at the top, and the function can be executed just by saying the function name in the middle of the program.
Function that returns a return value
Next, it is a type of program that returns a return value.
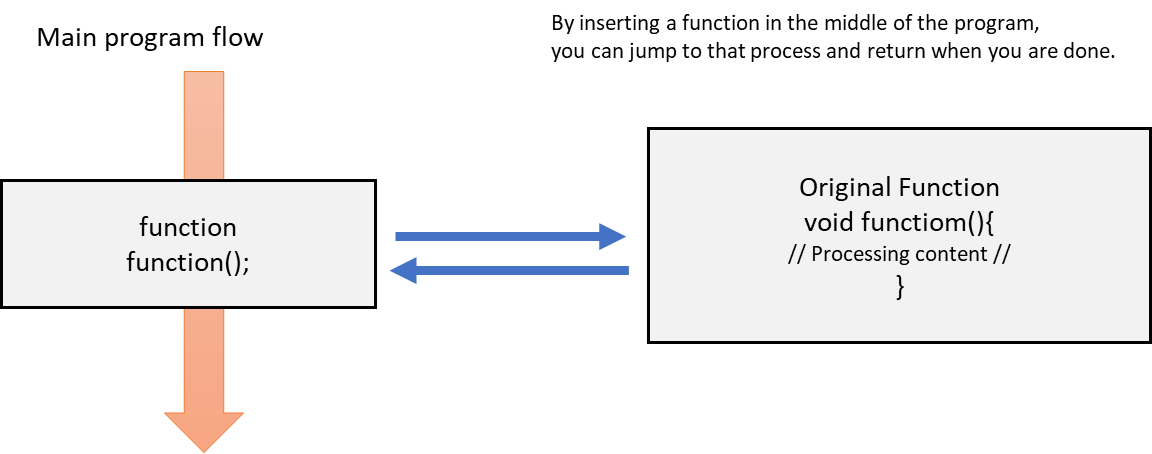
A type with a return value is, for example, to put a calculation formula in a function, send the parameter you want to calculate from the main program to the function, calculate it with the function, and return the calculation result as the return value.
In the example below, we want to make the velocity calculation a function.
Shows the definition of a program that returns a return value.
int function name (int variable 1, int variable 2, ...) {
What to do
Return return value variable;
}
Function name (variable 1, variable 2 ...);
Note that int can be double. Also, it doesn’t matter how many variables the feed value is.
From the main program, send the distance and time parameters to the function. It is calculated in the function and the calculation result (speed) is returned as the return value.
The following is an example program.
void setup() {
// put your setup code here, to run once:
Serial.begin(9600);
}
int sokudo(int d, int s) {
int a;
a=d/s;
return a;
}
void loop() {
// put your main code here, to run repeatedly:
int d;
d=105;
s=100;
x=sokudo(d,s);
Serial.println(x);
}
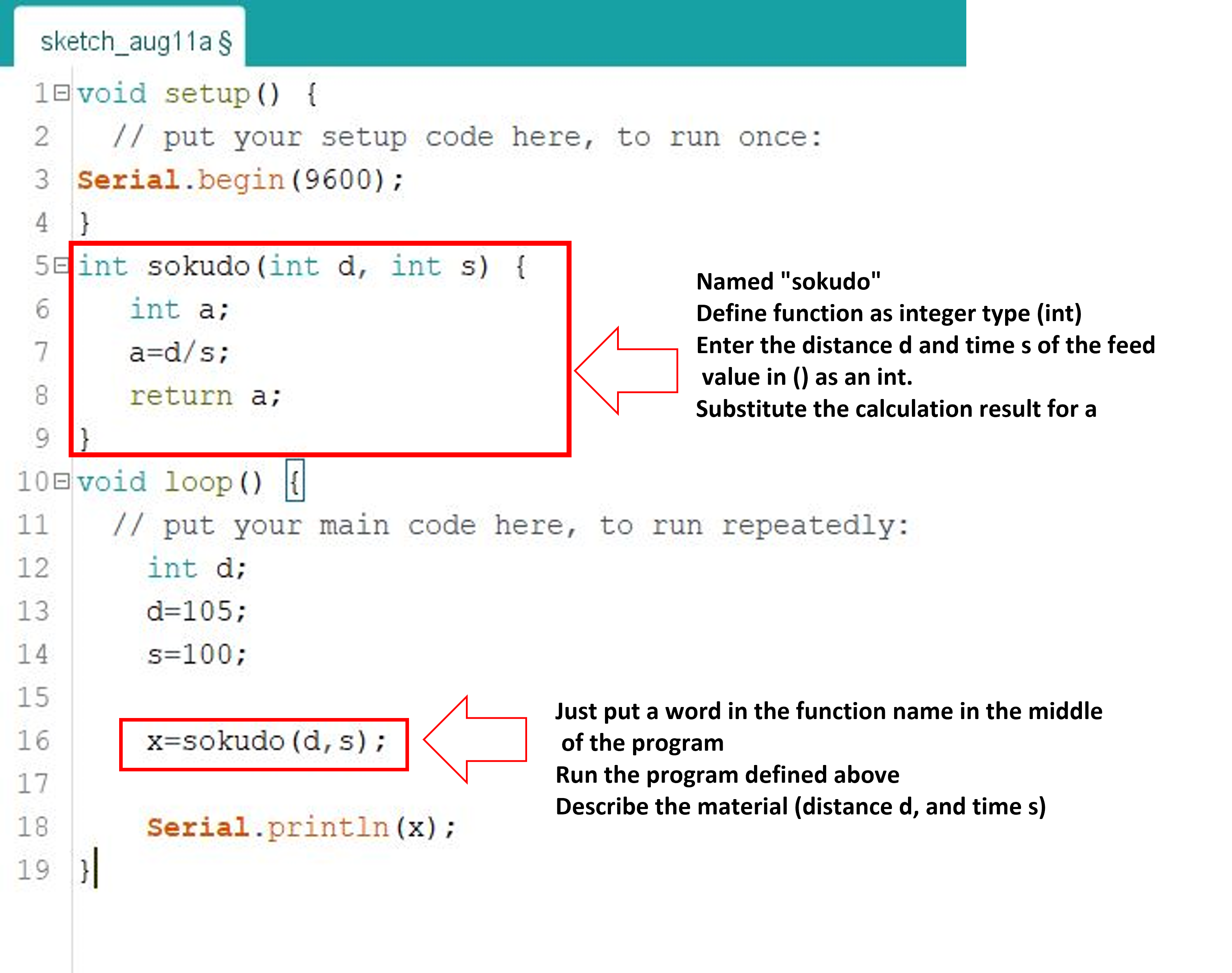
Define a function named sokudo as an integer type (int), describe the distance d and time s of the feed value in () as int, substitute the calculation result for a, and use return a; Returns as a return value.
The above is the rough contents of the function.
That’s all for today.